C++之数据类型……
信息在计算机中的表示
- 二进制数:0、1,称为一个bit(b)
- 八个二进制位称为一个byte(B)
$$
2^{10}B=1KB,,2^{10}KB=1MB,,2^{10}MB=1GB,,2^{10}GB=1TB
$$
整型
short至少16位
int至少与short一样长
long至少32位,且至少与int一样长
long long至少64位,且至少与long一样长
若不加usigned,默认为有符号整数
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
using namespace std;
int main(void)
{
//使用sizeof查看变量所占字节数
cout<<"int is "<<sizeof(int)<<" bytes"<<endl;
cout<<"short is "<<sizeof(short)<<" bytes"<<endl;
cout<<"long is "<<sizeof(long)<<" bytes"<<endl;
cout<<"long long is "<<sizeof(long long)<<" bytes"<<endl;
//通过climits库中的变量查看数据类型的最值
int n_int=INT_MAX;
short n_short=SHRT_MAX;
long n_long=LONG_MAX;
long long n_llong=LLONG_MAX;
cout<<"int max is:"<<n_int<<endl;
cout<<"short max is:"<<n_short<<endl;
cout<<"long max is:"<<n_long<<endl;
cout<<"long long max is:"<<n_llong<<endl;
return 0;
}结果如下:
char与cout.put()
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
using namespace std;
int main(void)
{
//char字符的使用
char ch='M';
int i=ch;
cout<<"The char is:"<<ch<<endl;//cin和cout的行为都是由变量类型引导的
cout<<"The ASCII code is:"<<i<<endl;
//使用cout对象中的.put()方法显示单个字符
cout.put('!');
return 0;
}结果如下:
溢出问题:
进制的表示与显示
- 十六进制:0x开头
- 八进制:0开头
1 |
|
结果如下:
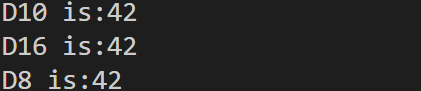
转义字符
浮点数
1.书写浮点数
使用常用的标准小数点表示
使用E/e表示法
2.浮点数的有效位数
C++有3种浮点类型:float、double、long double
float至少32位,double至少48位,且不少于float、long double至少和double一样多
float的精度(6~7位,其中6位一定是准的)比double低,尽量用double类型表示浮点数
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
using namespace std;
int main(void)
{
//浮点数的精度问题
cout.setf(ios_base::fixed,ios_base::floatfield);//强制cout显示小数点后6位
float tub=10.0/3.0;
const float million=1.0E6;
cout<<"tub= "<<tub<<endl;
cout<<"A million tubs= "<<million*tub<<endl;
cout<<"Ten million tubs= "<<10*million*tub<<endl;
double mints=10.0/3.0;
cout<<"tub= "<<mints<<endl;
cout<<"A million mints= "<<million*mints<<endl;
cout<<"Ten million mints= "<<10*million*mints<<endl;
return 0;
}结果如下:
3.浮点数的优缺点
优点:首先,它可以表示整数之间的值,其次,由于有缩放因子,它可以表示的范围大很多
缺点:浮点运算的速度通常比整数运算慢,且精度将降低
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
using namespace std;
int main(void)
{
//浮点数的缺点
float a=2.34E22;
float b=a+1.0;
cout<<"a="<<a<<endl;
cout<<"b="<<b<<endl;
cout<<b-a<<endl;
return 0;
}结果如下:
强制类型转换
(typeName) value
typeName (value)
实例1:对输入的整数,求其对应的天数小时分钟秒数
1 |
|
结果如下:
数组
- 数组中每个元素必须是相同的数据类型
- 计算机在内存中依次存储数组的各个元素
1.数组的声明
typeName arrayName[arraySize]
;- arraySize必须是整数常量或const值或常量表达式
2.数组的初始化
int cards[4]={3,6,8,10}
- 如果只对数组的一部分进行初始化,则编译器将把其他元素设置为0
short things[]={1,5,3,8}
//编译器将使things数组包含4个元素unsigned int couts[10]={}
//若不在大括号内包含任何东西,编译器将把所有元素都设置为零
3.矩阵乘法的实现
1 |
|
结果如下:
1 | 输入: |
字符串
1.字符串的创建
C-风格字符串:以‘\0作为字符数组的结尾
char dog[8]={'f', 'a', 't', 'e', 's', 's', 'a', '\0'}
char fish[]="Bubbles"
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
using namespace std;
int main(void)
{
const int size =15;
char name1[size];
char name2[size]="C++owBoy";
cout<<"Howdy! I am "<<name2<<". What your name?"<<endl;
cin>>name1;
//strlen()只计算字符串的长度,不包括空字符
cout<<"Your name has "<<strlen(name1)<<" letters"<<endl;
//sizeof()计算的是字符串数组所占据的字节数,共有15个char类型,每个char占一个byte
cout<<"Your name take up "<<sizeof(name1)<<" bytes"<<endl;
return 0;
}结果如下:
使用string类创建字符串
1
string name="ssyttmsl";
2.字符串的输入
cin.getline(variable,size)
读取一行输入,直到到达换行符,然后将换行符丢弃
相比于直接cin,可以读取空格,否则cin遇到空格则会停止读取
其中的第二个参数为size,最多存放size-1个字符
对于string类型的字符串,输入为
getline(cin,str)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
using namespace std;
int main(void)
{
/*使用getline()读取一行数据*/
const int size =15;
char name1[size];
char name2[size]="C++owBoy";
cout<<"Howdy! I am "<<name2<<". What your name?"<<endl;
cin.getline(name1,size);
//strlen()只计算字符串的长度,不包括空字符
cout<<"Your name has "<<strlen(name1)<<" letters"<<endl;
//sizeof()计算的是字符串数组所占据的字节数,共有15个char类型,每个char占一个byte
cout<<"Your name take up "<<sizeof(name1)<<" bytes"<<endl;
return 0;
}结果如下:
cin.get(variable,size)
- 读取一行输入,将换行符保留在输入序列中
- 直接使用cin也会读取换行符
- 使用不带任何参数的
cin.get()
,可读取下一行的字符(即使是换行符)
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
using namespace std;
int main(void)
{
/*使用get()读取一行数据*/
const int size =15;
char name[size];
char play[size];
cout<<"Please enter your name:";
cin.get(name,size);
cout<<"Please enter the sport you love:";
cin.get();//读取换行符
cin.get(play,size);
cout<<name<<" loves "<<play<<endl;
return 0;
}结果如下:
3.字符串的赋值、拼接和附加
对于string类型的字符串:
- 赋值:一个string对象可以赋给另一个string对象,但char数组却不行
- 拼接:使用运算符
+
将两个string对象合并起来 - 附加:使用运算符
+=
将字符串附加到string对象的末尾
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
using namespace std;
int main(void)
{
/*string的赋值、拼接、附加*/
string str1="ssy";
string str2;
//赋值
str2=str1;
cout<<"The str2 is:"<<str2<<",which is equal to str1:"<<str1<<endl;
//拼接
str2=" tql";
cout<<"The str1+str2 is:"<<str1+str2<<endl;
//附加
str1+=" is a excellent person.";
cout<<str1<<endl;
return 0;
}结果如下:
对于C风格的字符串
- 赋值:
strcpy(charr1,charr2)
//将字符串数组charr2赋给charr1 - 附加:
strcat(charr1,charr2)
//将字符串数组charr2添加到charr1的末尾
- 赋值:
4.计算字符串的大小
- 对于C风格的字符串,使用
strlen()
- 对于string类型的字符串,使用
str.size()
结构体
1.结构体的创建及其初始化
1 |
|
结果如下:
2.结构体数组
- 数组中的每一个元素都是结构体
1 |
|
结果如下:
共用体
- 共用体每次只能存储一个值,因此它必须有足够的空间来存储最大的成员,故共用体的长度为其最大成员的长度
1 |
|
结果如下:
枚举
- 枚举类型只有赋值运算,没有其他算数运算
- 只能对枚举类型赋予枚举中的值
- 枚举量是整形
- 默认枚举中的第一个数为0,后面的数依次+1
1 |
|
结果如下:
指针
1.指针的定义与使用
1 |
|
结果如下:
形象解释取地址&与*p:
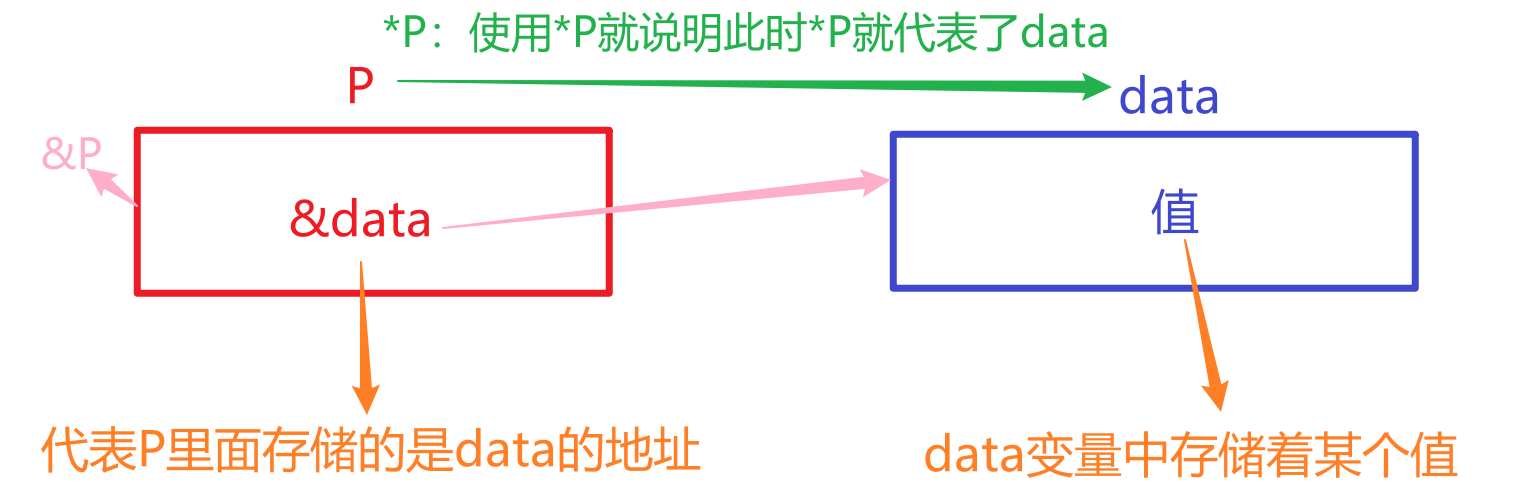
2.内存分配:new与delete
1 |
|
结果如下:
3.使用new来创建动态数组
1 |
|
结果如下:
形象解释:
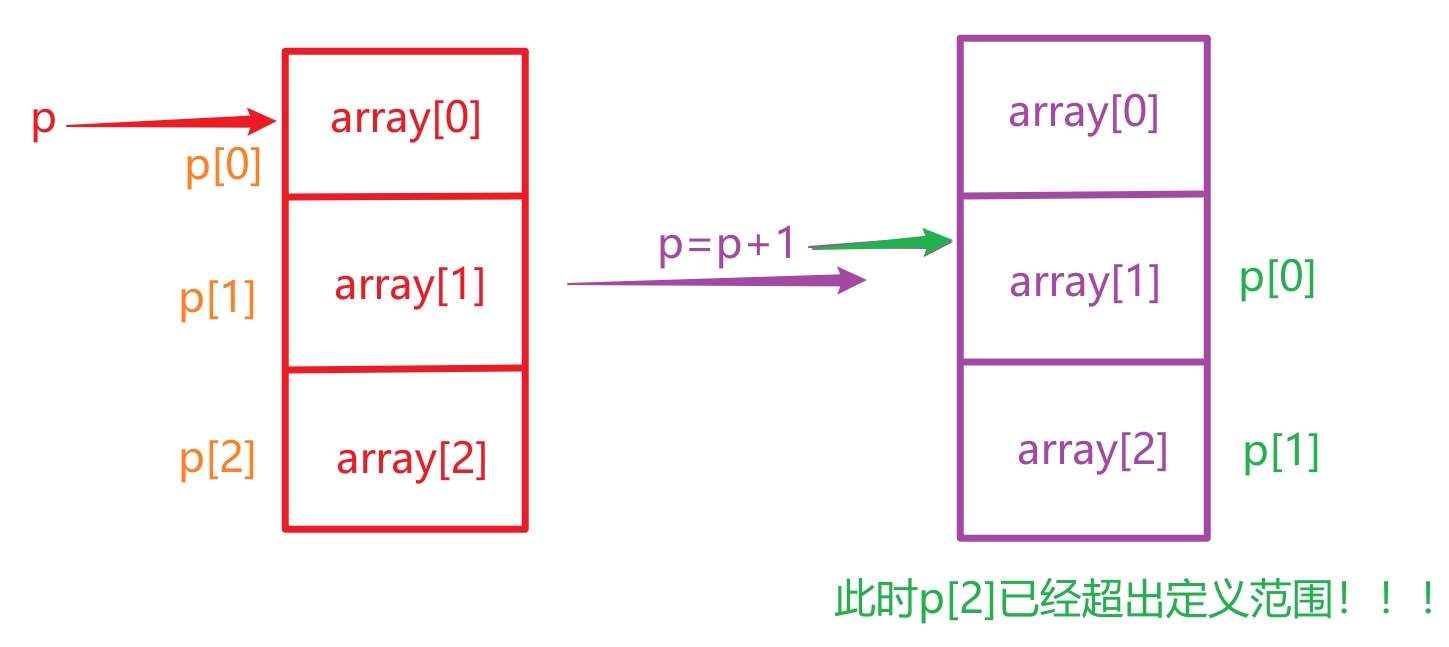
- 动态获取输入字符串
1 |
|
结果如下:
4.指针与数组
数组名
通常代表数组中第一个元素的起始地址&数组名
代表整个数组的起始地址- 其二者的区别在于它们+1时,前者加的是元素所占的字节数,后者加的是整个数组所占的字节数
1 |
|
结果如下:
5.指针与字符串
- C-type类型的字符串的数组名虽代表第一个字符的首地址,但打印时却会打印出地址对应的整个字符串
- 可通过
(int *)
将数组名强制转化成整数指针输出,从而打印地址
1 |
|
结果如下:
6.使用new创建动态结构
- 使用
p->变量
访问结构体中的变量 - 或者使用
(*p).变量
访问结构体中的变量
1 |
|
结果如下:
7.结构体数组与结构体类型的指针数组
1 |
|
结果如下:
形象解释:
8.指针与const
const int *pt;
//不能通过指针去改变指针所指向变量的值,但能指针所指向的地址可以改变,即指针自身所存储的内容可以被改变int *const pt;
//能通过指针去改变指针所指向变量的值,但不能改变指针中存储的内容,也就是指针一旦指定,就不能被改变去指向别的地址const int *const pt;
//结合上述二者,即不能通过指针去改变指针所指向变量的值,也不能改变指针里的内容
模板类vector与array
- 模板类vector的调用格式:
vector<typeName> vt(n_elem);
- 模板类array的调用格式:
array<typeName,n_elem> arr;
- 需要包含的头文件有
<vector>
与<array>
1 |
|
结果如下: